Send mail through SendGrid Web API using PowerShell
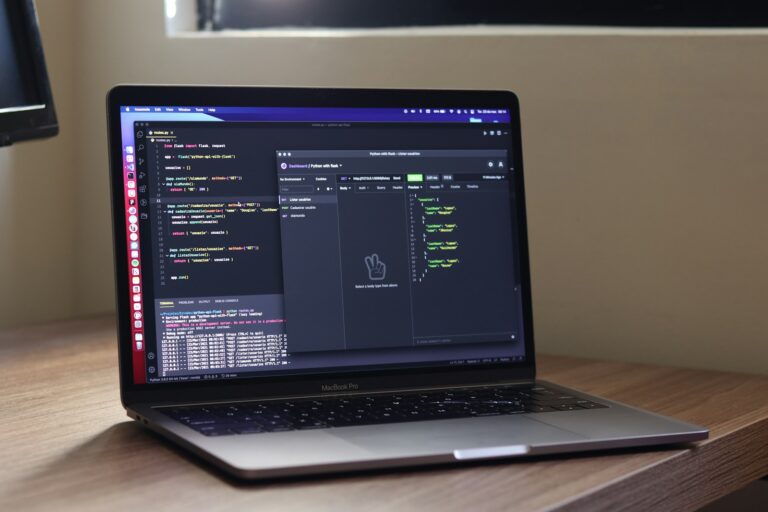
I found the SendGrid API documentation is lacking an simple PowerShell example to use their API, so here it is: Use Twilio SendGrid Web API v3 endpoints, including the new...
I found the SendGrid API documentation is lacking an simple PowerShell example to use their API, so here it is: Use Twilio SendGrid Web API v3 endpoints, including the new...
The Baidu spider (BaiduSpider user agent) can be a real pain to block, especially since it does not respect a robots.txt as it should. This post shows you how to...
The maximum length of a URL in the address bar is 2048 characters. In Windows Server IIS, its length is defined by the HttpRuntimeSection.MaxUrlLength property with a maximum length of...
Users can install and run multiple .NET Framework versions on their computers. When you develop or deploy your app, you might need to know which .NET versions are installed on...
There are a lot of hints & tips out there for troubleshooting SPNs, or Service Principal Names. Listing duplicate SPNs is fairly easy, just use setspn -X on your command...
Windows Server IIS is known for reusing DH key values, but there is a way to disable ECDH public server param reuse in Windows Server IIS. Here is how. Learn...
Disk cleanup in Windows Server using DISM is one of the most popular posts here on Saotn.org. It is still valid for Windows Server 2016 and up. So apparently, disk...
No more need for Windows Server IIS? Want to remove IIS completely from Windows Server using PowerShell? Learn how to remove IIS from Windows Server using PowerShell. Follow this if...
Sometimes you have use a specific TLS/SSL certificate or thumbprint for outgoing HTTPS connections because of endpoint restrictions. To test these connections you can use PowerShell, but how do you...
Easily send your DevOps reporting by email with this PowerShell function, because the Send-MailMessage cmdlet is obsolete. Of course you’ll be using StartTLS and authenticated SMTP as additional security. If...
Enabling Windows Defender per GPO failed with an error message “Get-MpComputerStatus : The extrinsic Method could not be executed.“, here is how to resolve this issue. Photo by Ed Hardie...
You can turn off Windows Defender routine remediation using a GPO setting called “DisableRoutinelyTakingAction”. This policy setting allows you to configure whether Microsoft Defender Antivirus automatically takes action on all...
Do you have a need for IIS in your Windows 11 development station, and now you wonder how to install IIS? Internet Information Services (IIS) for Windows Server is a...
Turn Windows Features on or off, Internet Information Services selected
Run PowerShell (PWSH) as Administrator
If you are using Windows Server IIS as your web server software, it is important to make regular backups. Luckily, using appcmd or PowerShell, this is quite easy. This post...
A quicky for my archives, learn how to convert decimal to hex and hexadecimal to decimal, in Bash… You can use your Linux Bash shell to easily convert decimal values...
Bash logo
About Jan Reilink The life and times of Jan Reilink Husband, father First and most important, I’m a husband and happily married to Lianne, and father of two great daughters:...
Windows Server 2016 was finally released last week, meaning we can finally lift the idiotic 260 characters limitation for NTFS paths. For this, you use a GPO or Group Policy...