Sometimes you need to convert a Windows SID (security identifier) to an username, or vice versa (username to SID). In this post I show you a couple of methods to translate the one into the other using VBScript and PowerShell.
Please note this post is a repost of a real old one (WaybackMachine mirror). I added some additional info, because it still comes in handy sometimes.
Username to Security Identifier (SID) VBScript example
VBScript? Yikes! For the old-school admins amongst us, here is an VBScript example to lookup an SID by the username. The VBS code utilizes WMI, that's fine because it's something we're familiar with.
Option explicit
Dim objAccount, strComputer, strUser
Dim objWMIService
If WScript.Arguments.Count < 1 Or WScript.Arguments.Count >= 3 Then
Wscript.Echo ""
Wscript.Echo "No or too many arguments provided."
Wscript.Echo "Usage: cscript nametosid.vbs NAME COMPUTER"
Wscript.Quit
Else
strUser = WScript.Arguments(0)
If Wscript.Arguments.Count = 2 Then
strComputer = LCase(Wscript.Arguments(1))
Else
strComputer = "."
End If
End If
Set objWMIService = GetObject("winmgmts:\\" & strComputer & "\root\cimv2")
Set objAccount = objWMIService.Get ("Win32_UserAccount.Name='" & strUser & "',Domain='" & strComputer & "'")
Wscript.Echo ""
Wscript.Echo strUser & " sid: " & objAccount.SID
Wscript.Echo ""
Set objAccount = Nothing
Set objWMIService = Nothing
Run this on your cmd.exe
command prompt:
cscript.exe /nologo nametosid.vbs name computername
Leaving out the computername may error out with the message: nametosid.vbs(21, 1) SWbemServicesEx: Not found.
Username to SID in PowerShell
There are two (well, more...) ways of translating an username to SID in PowerShell, one for domain users (where you have an Active Directory domain), and one for local users:
$objUser = New-Object System.Security.Principal.NTAccount("DOMAIN_NAME", "USER_NAME")
$strSID = $objUser.Translate([System.Security.Principal.SecurityIdentifier])
$strSID.Value
$objUser = New-Object System.Security.Principal.NTAccount("LOCAL_USER_NAME")
$strSID = $objUser.Translate([System.Security.Principal.SecurityIdentifier])
$strSID.Value
These use the System.Security.Principal.NTAccount class of .NET. Using Get-CimInstance / WMI, you can use:
Get-CimInstance -ClassName Win32_UserAccount | ?{$_.Name -eq "USER_NAME"} | Select-Object SID
#
# or in one go:
# (Get-CimInstance -ClassName Win32_UserAccount | ?{$_.Name -eq "USER_NAME"}).SID
But what if you want the username belonging to an SID? Translate SIDs to usernames with VBScript and PowerShell:
SID to username with VBScript
A short script to translate security identifier (SID) to a username in VBScript is:
Option explicit
Dim strSID
strSID = WScript.Arguments(0)
Dim strComputer
strComputer = "."
Dim objWMIService, objSID
Set objWMIService = GetObject("winmgmts:\\" & strComputer & "\root\cimv2")
Set objSID = objWMIService.Get("Win32_SID='" & strSID & "'")
WScript.Echo objSID.SID & ": " & objSID.AccountName
Set objSID = Nothing
Set objWMIService = Nothing
Run this in your cmd.exe shell, and it'll print out both the SID and the accompanying username:
cscript.exe .\sidtoname.vbs SID .
Use PowerShell to convert SIDs to usernames
Nowadays, we'd better use PowerShell for the job. In PowerShell you can also use WMI or utilize the System.Security.Principal.SecurityIdentifier .NET class to translate an SID into an NTAccount value. For example the following one-liner:
(New-Object System.Security.Principal.SecurityIdentifier("SID")).Translate([System.Security.Principal.NTAccount]).Value
Replace SID with the SID you want to convert. It'll write out the username. For WMI use:
Get-CimInstance -ClassName Win32_UserAccount | ?{$_.SID -eq "SID"} | Select-Object Name
#
# or in one go:
# (Get-CimInstance -ClassName Win32_UserAccount | ?{$_.SID -eq "SID"}).Name
Get-LocalUser and Get-ADUser PowerShell cmdlets
If you want to use "pure" PowerShell cmdlets, and not WMI or .NET classes, then you can always resort to the Get-LocalUser and Get-ADUser PowerShell cmdlets. A quick example of both:
Get-LocalUser | ?{$_.Name -eq "Jan Reilink" } | Select-Object SID
SID
---
S-1-5-21-3839202765-3620038548-2213037064-1002
# this one is fabricated, I'm not in an AD network
Get-AdUser -Identity "Jan Reilink" | Select-Object SID
SID
---
S-1-5-21-3839202765-3620038548-2213037064-1002
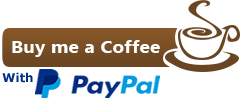
Thank you very much! <3 ❤️
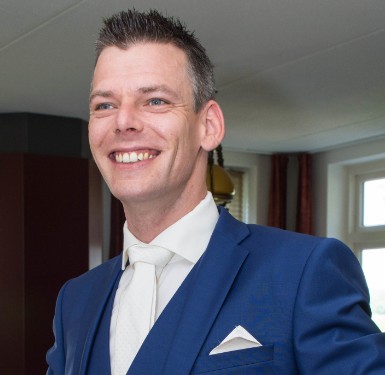
Hi, my name is Jan. I am not a hacker, coder, developer or guru. I am merely an application manager / systems administrator, doing my daily thing at Embrace – The Human Cloud. In the past I worked at CLDIN (CLouDINfra) and Vevida. With over 20 years of experience, my specialties include Windows Server, IIS, Linux (CentOS, Debian), security, PHP, websites & optimization. I blog at https://www.saotn.org.
Pingback: List all SPNs used in your Active Directory